If your company is looking to hire a truly great developer for your Android apps or if you’re applying for the role of an Android developer, how do you ensure that you can get the right candidate or are prepared for the job? It all starts with the Android interview questions. Going it alone is always a tricky business, someone can appear great in interview, but when stuff gets real they can sometimes be a disappointment. In this article we will explain how to separate the good from the not so good and ensure that your company ends up with the best candidates possible to fill this important role.
Interview techniques work both ways. As much as it is important for prospective candidates to develop good interview skills, so it is equally as important for interviewers to ask the right questions and develop skills to avoid hiring the wrong person. Confidence comes from having the right ammunition in your locker. It’s all about understanding the position, the requirements for the job and the necessary questions in order to ensure that the interviewee is suitable.
During the Interview
It is important that you make the interviewee feel at ease. Nobody truly enjoys the interview process, and it can be extremely stressful. The more that you can do to put the candidate at ease, the more likely you are to get a genuine feel for their character. Obviously as with any candidate for any position in your company the basic questions to determine their character and past working practices need to be addressed. It is a good idea to check out social media as well. These days everyone has a social media presence and it’s a good idea to take a quick look at their posting record. If they show a lack of integrity towards previous employers there is no reason to suggest that they will suddenly change their behavior patterns. For more info on how to choose the right developer for you company, visit our blog post here. So after you have asked the usual generic questions and made the candidate feel comfortable, it’s time to find out just how much they know about Android developing. Here’s where the questions get more specific to the job and maybe even more difficult.In case you’d like to skip ahead…
Essential Questions
1. What is Android and who founded it?
Android is an open-source, linux-based operating system. It was founded by Andy Rubin and it is used in mobiles, tablets, televisions etc.
2. Please name the Android Application Architecture.
- Activities dictate the UI and handle the user interaction with a smartphone screen. Activity performs actions on the screen.
- Broadcast Receivers respond to broadcast messages from other application in or from the system. This is implemented as a subclass of BroadcastReceiver class and each message is recognized as an Intent object.
- Services. These are used to perform background functions.
- Intent. This is what enables inter connectivity between activities and data passing mechanism.
- Resource Externalisation which refers to strings and graphics.
- Notification for dialogue box, icon, light, notification, sound and toast
- Content Providers for sharing data between applications
3. What are the additional components of Android?
- Fragments serve as a portion of user interface in an Activity.
- Views are UI elements that are drawn on-screen including buttons, lists forms, etc.
- Layouts view hierarchies that control screen format and the appearance of the views.
- Intents are messages that wire components together.
- Resources are external elements (strings, constants and drawable pictures).
- Manifest is the configuration file for the app.
4. What notifications are available in Android and what is their usage?
Snackbars & Toast Notification − Shows up as a pop up message on the surface of the window.
Snackbars contain a single line of text that is directly relation to the operation that is performed. They typically contain a text action and no icons. Only one snackbar can be displayed at a time and it can contain a singly action, neither of which may be “Dismiss” or “Cancel.”
Toasts are only available with Androids and they are used for system message. They also display at the bottom of the screen but they can’t be swiped off-screen.
Status Bar Notifications show notifications on the status bar.
For more information about Snackbars and Toast, visit here.
Dialogue Notification − An actively related notification.
5. How do you Translate in Android?
Android uses Google translator to translate data from one language into another language and places it as a string while development.
6. What types of flags are used to run an application in Android?
FLAG_ACTIVITY_NEW_TASK
FLAG_ACTIVITY_CLEAR_TOP.
7. Android Versions go under code names, please give as many of the code names as you know.
Aestro, Blender, Cupcake, Donut, Eclair, Froyo, Gingerbread, Honeycomb, Ice Cream Sandwich, Jelly Bean, Kitkat, Lollipop, Marshmallow
8. What are the main advantages of Android?
Android is an open source operating system which means that is is free to the end user. There are no license, development or distribution fees. It supports many different technologies including camera, bluetooth, wifi, speech, and edge. In addition it also utilises a highly optimised virtual machine called DVM (Dalvik Virtual Machine) for use on mobile devices.
9. Can you name the database which Android uses and give a brief description about it?
The name of the database is SQLite which is an open-source relational database. It can be used to perform the usual database functions on Android devices. Not only is it easy to store, manipulate and retrieve data but it is also embedded into the Android platform as a default. No setup is necessary, the administration is already in place.
10. What are the different types of storages that are available in Android and what is their usage?
- Shared Preferences store private primitive data in key-value pairs. SharedPreferences class helps to provide a general framework that allows users to save and retrieve persistent key-value pairs of primitive data types. SharedPreferences can be used to save primitive data such as booleans, floats, ints, longs and strings. This data persists across user sessions even when one’s application is killed. For more information on how to get a SharedPreferences object for one’s application click here.
- Internal Storage stores private data on the device memory. Once can save files directly on the device’s internal storage. The files saved to the internal storage are private to you application by default and other applications cannot access them either. When the user uninstalls your application, the files will then be removed.
- External Storage stores public date on the shared external storage. Every Android-compatible device can support a shared “external storage” where one’s files can be saved. This can be removable storage media (SD card) or internal (non-removable) storage.
- SQLite Databases stores structured data in a private database. Android provides full support for SQLite databases. Any databases that one creates can be accessible by name to any class in the application. Please note they are not accessible outside of the application.
- Network Connection stores data on the web with one’s own network server. In order to do network operations, one must use classes in the following packages below:
11. What are application Widgets in Android?
Application Widgets are miniature application views that can embedded in other applications (such as the Home screen) and receive periodic updates. These views are often referred to as Widgets in the user interface, and you can publish one with an App Widget provider.
Building Blocks & Life Cycles
12. What are the Android core building blocks
The core building blocks for Android are as follows:
- Activity – The class representing a single screen, i.e. a Frame in AWT
- View – The UI element for example a label, button or text field. Basically anything that is seen is a view
- Intent – that which is used for invoking components. For example start the service, display a web page or broadcast a message.
- Service – the background process. These come in two types local which is accessed from the application and remote which can be accessed from other devices.
- Content Provider – these are used to share data between different applications.
- Fragment – separate parts of the activity which can be displayed in multiples at the same time.
- AndroidManifest.xml – This holds information regarding activities, permissions and content providers etc.
- Android Virtual Device (AVD) – Which is used for testing the application without need a tablet or other device.
13. Can you name the 7 life-cycle methods of Android activity and explain a little about each one?
The 7 life-cycle methods are as follows:
1) onCreate() – meaning that an activity has been created
2) onStart() – meaning it has become visible to the user.
3) onResume() – meaning that the activity has started interacting with the user
4) onPause() – meaning the activity is not visible to the user
5) onStop() – meaning it is no longer visible to the end user
6) onRestart() – meaning the activity has stopped, prior to being started
7) onDestroy() – meaning the activity will be destroyed
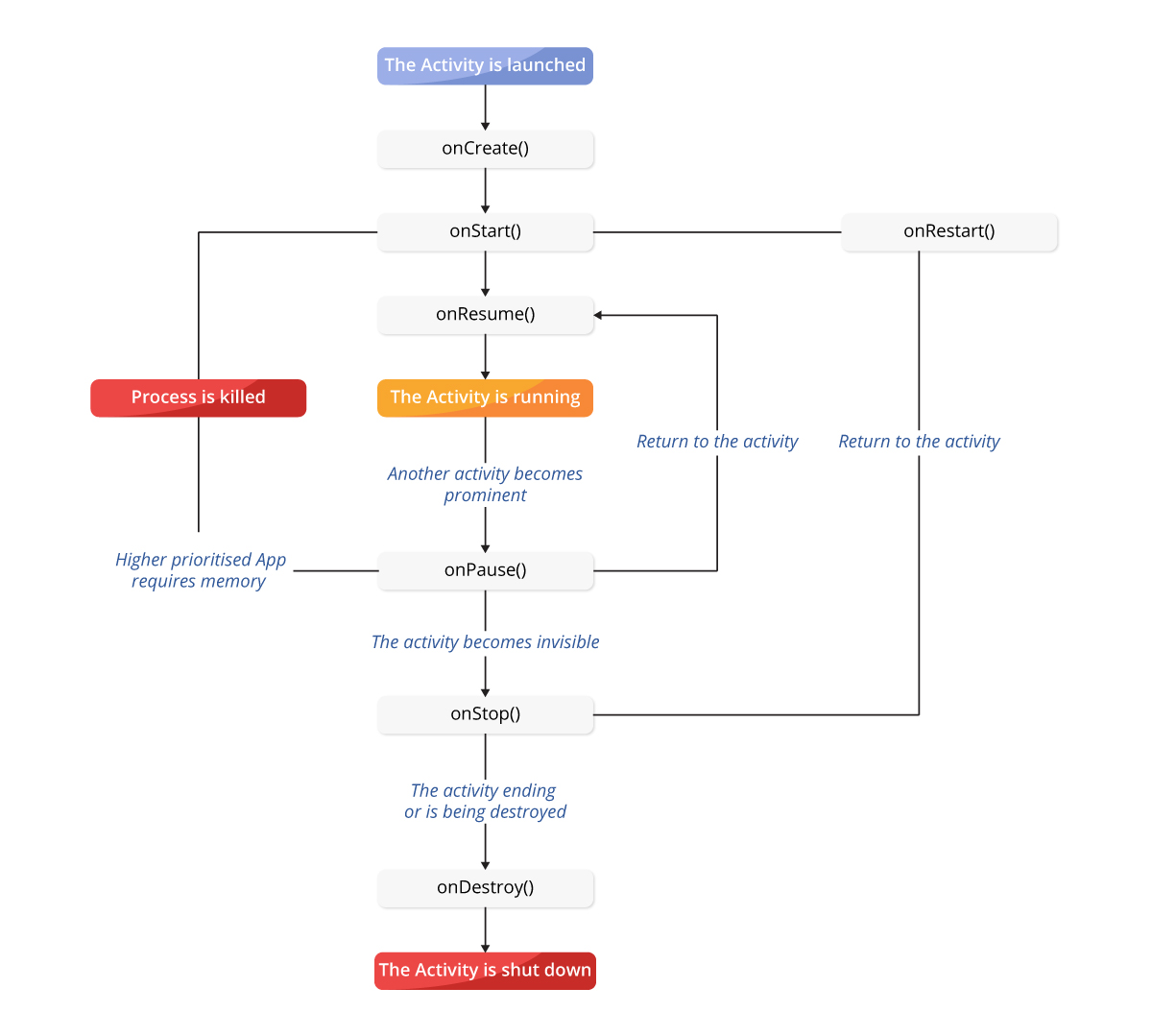
Android Acronyms
14. What does ADB in Android stand for?
ADB acts as a bridge between emulator and IDE. It also executes remote shell commands to run applications on an emulator.
15. What is ANR in Android?
ANR stands for application is not responding. It is a dialog box that appears when the application is not responding.
16. What does ADT stand for?
ADT is Android development tool and it is used to develop the applications and test the applications.
17. What does DDMS stand for and what are its capabilities
This refers to the Delvik Debug Monitor Server. Thesis a debugging tool that is included in Android Studio. It can be used for port-forwarding services, thread and heap information on the device, screen capture on the device, logcat, process, and radio state information. In addition it provides, among other services, incoming call and SMS spoofing, and location data spoofing.
For more information please read here.
Intent
18. What is an intent and give three examples of how it is used?
Three common uses for an intent are:
- Starting an Activity It is normal to start a new instance of an activity by passing an intent to startActivity() method.
- Delivering a Broadcast This can be done by passing an intent to sendBroadcast(), sendStickyBroadcast(), and sendOrderedBroadcast()
- Starting a Service this can be used to perform a one-time operation, for example download a file. It’s achieved by passing an intent to startService()
19. Can an intent be used to give data to a ContentProvider ?
No, an intent cannot be used to give data to a ContentProvider. In order to use data in a ContentProvider you have to use ContentResolver instead. This is in the application’s Context and is used to communicate with the provider as a client. The provider object works by receiving data requests, performing the required action and then returning the results.
20. Using with intent, we can launch an activity.
Intent intent = new Intent(this, MyTestActivity.class); startActivity(intent);
Definitions & Solutions
21. Define the application resource file in Android.
JSON,XML bitmap.etc are application resources.You can injected these files to build process and can load them from the code.
22. What is an Adapter in Android?
An Adapter acts as a bridge. It converts data items into new items so it can be displayed in UI components.
For more information about Adapters, visit here.
23. Where are layouts placed in Android?
In The Layout folder, layouts are placed as XML files.
24. What is a singleton class in Android?
A class that can create only an object, that object can be shared withl other classes.
25. What is a fragment in Android?
A fragment is a piece of activity and it is always contained in activity. With fragment, we can reuse it in many activities and it is more flexible to locate on a screen.
26. What is sleep mode in Android?
Sleep mode means that the CPU will be sleeping and it doesn’t accept any commands from an Android device except Radio interface layer and alarm.
27. Which kernal is used in Android?
Android is a customised Linux 3.6 kernel.
28. Which exceptions are available in Android?
InflateException,Surface.OutOfResourceException,SurfaceHolder.BadSurfaceTypeException,and WindowManager.BadTokenException
29. What is the order of the dialog-box in Android?
The order is: Positive, Neutral, Negative.
30. What is a drawable folder in Android?
A compiled visual resource that can used as a backgrounds,banners, icons,splash screen etc.
31. What does ContentProvider mean and what is it’s normal use?
A ContentProvider is used to manage access of a structured set of data. It identifies the data and provides mechanisms for defining the data security. It is the standard interface connecting data within one process with the code running in another. For more information please refer to this section of the Android Developer’s Guide.
32. How do you pass the data to sub-activities android?
Using with Bundle, we can pass the data to sub activities.
Bundle bun = new Bundle();
bun.putString(“EMAIL”, “contact@tutorials.com“);
33. You are reorienting a screen, instead of Android tearing down the foreground and restoring the view values in the activity’s layout, a view’s value is not restored after the reorientation. What is the likely reason for this?
The most likely reason is that the developer has not verified that it has a valid id. An Android system will only restore the state of views in the activity if each view is given a unique id. This is supplied by the attribute, android:id
For more information please refer to this section of the Android Developer’s Guide.
Android Library
34. Describe the Android library.
An Android library is a development project that holds shared Android source code and other resources. It holds everything necessary in order to build an app including source code, resource files, and an Android manifest. It compiles everything into an Android Archive (AAR) file that can be used as a dependency for an Android app module.
35. When is the Android library module used?
The Android library module can be used when one is building multiple apps that require or use the same components like activities, services, or UI layouts. It’s also used when one is building an app that exists in multiple APK variations such as free and paid version that need the same core components for both.
This guide can help users learn how to create an Android library.
In order to stay up-to-date with the latest Android developments, it’s important that you continue to develop your skills as the systems develop and change over time as well. This will allow you to stay ahead of the competition and become a more valuable asset to your client and your team.
Android Thread
36. What is a Thread?
“A thread is a thread of execution in a program.” Each thread has a priority and the higher priority the thread has they’re executed in preference to threads with lower priority. When code is running in thread and creates a new Thread object, the new thread has its priority initially set equal to the priority of the creating thread, and is a daemon thread if and only if the creating thread is a daemon.
37. How does one create a new thread of execution?
There are two ways that one can create a new thread of execution. One is by declaring a class to be a subclass of Thread. The subclass will override the run method of class Thread. The subclass can be allocated and started. An example of a thread that compuets primes larger than a stated value is below:
class PrimeThread extends Thread { long minPrime; PrimeThread(long minPrime) { this.minPrime = minPrime; } public void run() { // compute primes larger than minPrime . . . } }The code below would create a thread and start it running:
PrimeThread p = new PrimeThread(143); p.start();Another way to create a thread is to declare a class that implements a Runnable interface. This class will implement the run method. The class can be allocated, passed as an argument when creating Thread and started.
class PrimeRun implements Runnable { long minPrime; PrimeRun(long minPrime) { this.minPrime = minPrime; } public void run() { // compute primes larger than minPrime . . . } }The code below would create a thread and start running:
PrimeRun p = new PrimeRun(143); new Thread(p).start();
38. Can more than one thread have the same name?
Yes. Every thread has a name in order to be identified. If a name is not specified when a thread is created, a new name is created for it.
For more information about Android thread visit here.