Python is kind of trending language these days with its wide range of applications from science to web development and so on. If you’re looking for a job as a Python developer or if you’re interviewing candidates for a role on your team, this tutorial will come in handy.
Firstly, Python Developer means Software Developer specifically in Python language and its related Frameworks of working with a team.
We’ll divide this section of interviewing a Python developer into four parts:
- Common software engineering skills
- Python language specific questions
- Python related framework, library (related to the product/project we are working on)
- Non-technical questions
Common Software Engineering Skills
Common and important concepts
We’d recommend asking your candidates some important concepts in the realm of software engineering such as:-
Big-O:
def contains_duplicates(elements): for i, outer in enumerate(elements): for j, inner in enumerate(elements): # Don't compare with self if (i == j): continue if (outer == inner): return True return False
-
Common algorithms and data structures:
-
Debugging with an interactive debugger:
Relational and non-relational databases:
Database is an integral part of software. Here, we will ask the candidate to talk about the characteristics and benefits of relational and non-relational databases and when we should use them.-
Relational:
- Table
- record
- column
- relation
- index.
-
Non-relational databases:
A database that does not incorporate the table/key model that relational database management systems (RDBMS) promote. These kinds of databases require data manipulation techniques and processes designed to provide solutions to big data problems that big companies face. The most popular emerging non-relational database is called NoSQL (Not Only SQL).Go for NoSQL databases when there is no fixed (and predetermined) schema of data fits in. In NoSQL, Scalability, Performance (high throughput and low operation latency), Continuous Availability are very important requirements to be met by the underlying architecture of the database. NoSQL can store enormous amounts of data in the range of petabytes.
-
Database index
-
Automated Unit Testing
-
Version control
-
SOLID DRY KISS
-
Regular expression
-
Software Architecture and Design Patterns
Problem Solving Ability
During the interview process it’s important to evaluate your candidate’s ability to solve complex problems. Not simply if they can solve a problem but also to understand how they came to the solution. Ask the candidate to solve some quick problems so you can evaluate how the candidate approaches and solves each issue. As the interviewer, you should assessing how quickly they arrived to a good solution and how good that solution actually was. Below is a sample question: Given a string, find the length of the longest substring without repeating characters. Additional examples:- Given “abcabcbb”, the answer is “abc”, which the length is 3.
- With “bbbbb”, the answer is “b”, with the length of 1.
- Given “pwwkew”, the answer is “wke”, with the length of 3. Note that the answer must be a substring,
- “pwke” is a sub-sequence and not a substring.
def longest_substring(s): char_dict = {} longest_count = 0 for i, c in enumerate(s): if c in char_dict: longest_count = max(longest_count, len(char_dict)) dup_i = char_dict[c] char_dict = {k: v for k, v in char_dict.items() if v > dup_i} char_dict[c] = i return max(longest_count, len(char_dict))Additional questions similar to above can be found here.
Python Language Specific Questions
Question 1: What is Python and what are the key features of Python?
Some key points:- Python is a programming language with objects, modules, threads, exceptions and automatic memory management. The benefits of using Python are that it is simple and easy, portable, extensible, built-in data structure and it is an open source.
- Python is an interpreted language. That means that, unlike languages like C and its variants, Python does not need to be compiled before it is run. Other interpreted languages include PHP and Ruby.
- Python is dynamic but strong typing, meaning that you don’t need to state the types of variables when you declare them or anything along those lines.
- You can do things like x=1 and then x=”A string” without error. But when you manipulate variables with different types, it will generate errors, unlike C# or Java: print(“A” + 1) # TypeError: must be str, not int
- In Python, functions are first-class objects. This means that they can be assigned to variables, returned from other functions and passed into functions. Classes are also first-class objects. This is similar to Javascript.
- Python is used widely from web applications, automation, scientific modeling, big data applications and many more. But it is famous for machine learning and data science. Python is one of the most popular programming languages used by data analysts and scientists.
Question 2: What is dictionary in Python?
A dictionary is a collection which is unordered, changeable and indexed. In Python, dictionaries are written with curly brackets, and they have keys and values. It defines one-to-one relationships between keys and values. Dictionaries contain a pair of keys and their corresponding values. Dictionaries are indexed by keys. An important feature of a dictionary is the complexity of getting and setting an element is O(1). For example:new_computer={'Chipset':'8th-generation Intel Core i7 processor','Card':'Radeon Pro 560X','Ram':'16GB 2400MHz DDR4', 'Storage': '512GB SSD'} print(new_computer['Chipset']) '8th-generation Intel Core i7 processor'
Question 3: Looking at the below code, write down the final values of A0, A1, …An.
A0 = dict(zip(('a','b','c','d','e'),(1,2,3,4,5))) A1 = range(10) A2 = sorted([i for i in A1 if i in A0]) A3 = sorted([A0[s] for s in A0]) A4 = [i for i in A1 if i in A3] A5 = {i:i*i for i in A1} A6 = [[i,i*i] for i in A1]Answer:
A0 = {'a': 1, 'c': 3, 'b': 2, 'e': 5, 'd': 4} # the order may vary A1 = range(0, 10) # or [0, 1, 2, 3, 4, 5, 6, 7, 8, 9] in python 2 A2 = [] A3 = [1, 2, 3, 4, 5] A4 = [1, 2, 3, 4, 5] A5 = {0: 0, 1: 1, 2: 4, 3: 9, 4: 16, 5: 25, 6: 36, 7: 49, 8: 64, 9: 81} A6 = [[0, 0], [1, 1], [2, 4], [3, 9], [4, 16], [5, 25], [6, 36], [7, 49], [8, 64], [9, 81]]List comprehension is an important task developers with work with every day. Not only Python but also in many other languages. So, this is a must-ask question to see if the candidate is truly familiar with Python.
Question 4: How is memory managed in Python?
Memory management in Python is managed by Python private heap space. All Python objects and data structures are located in a private heap. The programmer does not have access to this private heap. The Python interpreter takes care of this instead. The allocation of heap space for Python objects is done by Python’s memory manager. The core API gives access to some tools for the programmer to code. Python also has an inbuilt garbage collector, which recycles all the unused memory so that it can be made available to the heap space.Question 5: What is monkey patching in Python?
In Python, the term ‘monkey patch’ only refers to dynamic modifications of a class or module at run-time. Consider the following example:# m.py class MyClass: def f(self): print "I am f()"We can then run the monkey-patch testing like this:
import m def monkey_f(self): print "I am monkey_f()" m.MyClass.f = monkey_f obj = m.MyClass() obj.f()The output will be I am monkey_f(). As we can see, we did make some changes in the behavior of f() in MyClass using the function we defined, monkey_f(), outside of the module m.
Question 6: What are negative indexes and why are they used?
The sequences in Python are indexed and it consists of the positive as well as negative numbers. If you know Ruby, it’s kind of similar. It can be compared to the way of counting forward and backward in a circle. The index for the negative number starts from ‘-1’ that represents the last index in the sequence and ‘-2’ as the penultimate index and the sequence carries forward like the positive number. The negative index is used to remove any new-line spaces from the string and allow the string to except the last character that is given as S[:-1]. The negative index is also used to show the index to represent the string in the correct order.Question 7: What is the difference between range and xrange?
In Python 2, range creates a list, so if you do range(1, 10000000) it creates a list in memory with 9999999 elements otherwises xrange is a sequence object that lazily evaluates. But in python3, range does the equivalent of Python’s xrange.Question 8: Consider the following code, what will be its output?
class A(object): def go(self): print("go A go!") def stop(self): print("stop A stop!") def pause(self): raise Exception("Not Implemented") class B(A): def go(self): super(B, self).go() print("go B go!") class C(A): def go(self): super(C, self).go() print("go C go!") def stop(self): super(C, self).stop() print("stop C stop!") class D(B,C): def go(self): super(D, self).go() print("go D go!") def stop(self): super(D, self).stop() print("stop D stop!") def pause(self): print("wait D wait!") class E(B,C): pass a = A() b = B() c = C() d = D() e = E() # specify output from here onwards a.go() b.go() c.go() d.go() e.go() a.stop() b.stop() c.stop() d.stop() e.stop() a.pause() b.pause() c.pause() d.pause() e.pause()Answer:
a.go() # go A go! b.go() # go A go! # go B go! c.go() # go A go! # go C go! d.go() # go A go! # go C go! # go B go! # go D go! e.go() # go A go! # go C go! # go B go! a.stop() # stop A stop! b.stop() # stop A stop! c.stop() # stop A stop! # stop C stop! d.stop() # stop A stop! # stop C stop! # stop D stop! e.stop() # stop A stop! a.pause() # ... Exception: Not Implemented b.pause() # ... Exception: Not Implemented c.pause() # ... Exception: Not Implemented d.pause() # wait D wait! e.pause() # ...Exception: Not ImplementedBecause OO programming is extremely important in today’s industry. Correctly answering this type of question shows that your candidate understands the inheritance and the use of Python’s super function.
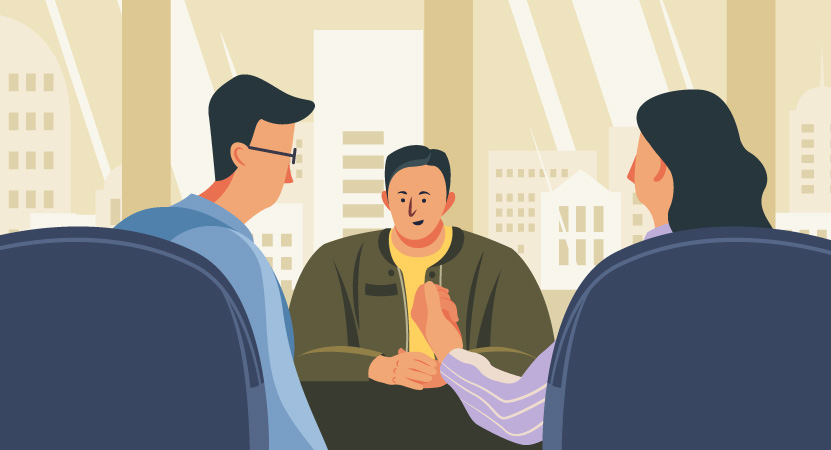
Conclusion
And there you have it. The topics covered in this tutorial will help you to separate the experienced from the not so experienced while interviewing candidates to join your team as well as provide strong preparation material for those interviewing for a Python developer role.
Tagged pythonpython developer