Google “What is Ruby” and below you’ll find the most common representation.
Ruby is an object-oriented programming language that uses a model-view-controller (MVP) architecture.
Although the folks that used Ruby to build Twitter probably knew more than just the basics. It’s completely possible to use Ruby to build something as tremendous as Twitter and not know a single lick of code. While you may want to do a deeper dive on your way to building the next world-changing social media platform, getting started—and even delivering your first app—might be something you can do before sundown.
In this post we’ll go over:
This command sets up your blog and puts it online on a Rails server instantly. Congratulations, you’ve made your first foray in to being a Ruby on Rails developer.
- What is Ruby and why you want to develop within Ruby on Rails
- Why not knowing Ruby or any code at all is an advantage
- What programs you’ll need to get started
- Ruby projects that you can use to learn and developer your Ruby skills
To Write Ruby You Don’t Need To Write Ruby Per Se
The easiest way to get started with Ruby is to learn via Ruby on Rails. While Ruby is code, Ruby on Rails is not. Rather, it’s a tool that pieces together various prefabbed Ruby functions and presents everything in a friendly, easy-to-use interface in which you can create web-ready Ruby programs. This differs from conventional programming in that you’re not really programming. There are some key terms to know (first and foremost among them the “model-view-controller” scheme mentioned above) and there is a certain “grammar” to Rails, but it looks and feels like something closer to actual, literal plain English.Model-View-Controller
In TK’s blog on Medium regarding Ruby on Rails programming, he calls the MVC or Model-View-Controller scheme a “separation of the concerns” as a way to describe how Ruby on Rails delegates responsibilities among its discreet parts.Model
This is the part of Ruby on Rails where the programming defines the relationship between an object and a database. For example, when the object has a transactional relationship with the database—”if object does X, then database responds with Y”—the model describes what occurs. Curiously (or perhaps confusingly) the model itself exists as an object. This model object is the layer between your application and the relevant databases.View
This aptly named category of Ruby code defines what the user will view inside your Ruby app or program. This is what delivers Ruby’s user interface. A “view” request can summon forth a PDF or a piece of HTML if it’s a webpage.Controller
Governing the structure is the controller. As a browser moves through the Internet and finds your Ruby-enabled app or website, the first thing it interacts with is the controller, which will tell the models what pieces of the database to activate and to recall. It also liaises between this activity and the view. The view kicks a user interface out to the browser and viola, that’s what you see on the screen.Putting it all together
In practice, how this looks is that a user makes a request to a computer server by, say, typing in a URL or updating a newsfeed on their app, and this triggers an interaction with a Ruby on Rails application held on the server. Ruby on Rails matches the user request—whether it’s seeing all the latest articles, or checking the status of a shipping order—with the right controller, which cues a model to query a database and deliver a server response, the view, the user interface and just everything else you might see, hear or experience on the client side.Ruby vs. Ruby on Rails
The preposition in “Ruby on Rails” is probably one of the most helpful ways in understanding the difference between the programming language Ruby and the batched functions known as Rails. Ruby is a programming language like Perl or C++ that uses symbols and syntax to create programs. It’s generally described as an easy-to-learn, powerful programming language that makes sense in reading it. Lauding notes aside, if you’re trying to work with Ruby to build an application from scratch, the learning curve is pretty steep. It is after all a programming language. But what if you could automate some of the more routine parts of application building? What if for example you could just type in “make a blog” and the code would respond with “enable posting,” “enable editing,” and all the things you just assume are part of a blog function. This is a fair approximation of what Rails is. Rails is a web application that has a lot of pre-made functions and tools that enable you to build quick and, in many ways, better code that is more functional and easier to maintain than without Rails. Rails is web-centered; it’s been described as the “glue” that holds Rails and the Internet together. If a metaphor would make it easier to understand, imagine you’re hungry and Ruby is like growing all the food yourself and building your own fire. Also, you eat with your hands. Rails is like being hungry and being able to make a meal from a stocked pantry inside of a home furnished with a stove and cooking utensils. Also, you have a great live-in significant other to share the meal with and a dog that will eat whatever you drop.Ignorance is Your Edge
But in turn, not knowing a programming language may actually be an asset to you as a beginning Ruby on Rails developer. Because Ruby on Rails is built in a plain language-like grammar, there are meaningful differences with how you write in Ruby on Rails versus another programming language like PHP. Using these two languages as an example, take how they both approach ending a line of code. Within PHP, you’d use the curly brackets or { } to open and close blocks of code. In Ruby on Rails by contrast, you just type in “end.” If the above seems like just a superficial difference, let’s look at a deeper, more feature-specific difference like how very different it is to get the time a month from now in Ruby on Rails versus Python as explained by code learning blog One Month. To get the time one month from now in Ruby on Rails, you use these two command lines:require8 'active_support/all' new_time = 1.month.from_nowThe active support extensions you see above are part of the Ruby on Rail component that is “responsible for providing Ruby language extensions, utilities, and other transversal stuff.” It also imports a library of functions that make Rails recognize the “.month” and “.from_now” functions. You can find a more in-depth guide to active support core functions here. Ruby on Rails is often described as “magical,” which is closer to criticism than it is praise. Deep understanding of how the code works is hard and not even really required if you just know which part of it to use and for what. Ruby on Rails is built on the understanding that you need functionality more than you need to acquire a deep knowledge of code. To do the same thing in Python, you have to use a very different set of commands that do a very different set of things. Are you still with us?
from datetime import datetime from dateutil.relativedelta import relativedelta new_time = datetime.now() + relativedelta(months=1)The line of Python code above, by contrast, is almost humorously explicit. Two function imports happen from “datetime” and “relativedelta” databases; that creates the date and time data and then the second creates past or future time and data. Time plus change equals new time. Less magical but more direct. There are great differences between computer language and spoken language, but here, it’s worth thinking about the two analogously. The programmers who are intimate with Python or another programming language have had to learn it similar to the way you’d learn a spoken language, with rules that get baked in after many months or years of working with it. The Python or Perl programmer pivoting to Ruby on Rails is in some respects like the English language learner. While some core concepts are similar about the language they’re coming from, Ruby on Rails is simply different in the way that French and English are different. They say babies have an easier time learning languages. Perhaps some of that is due to their not being burdened with previously held rules and ways of thinking and doing. Their ignorance is their edge. If you’ve never programmed before, this is similarly your situation.
Installing and Working With Ruby on Rails
Ruby on Rails works on both Windows and MacOS, and there are plenty of reliable websites that offer both versions like this one for Apple machines and this one for Windows. Ruby on Rails is not a program itself, but a bundle of different programs including the MySQL database, Git, Rails and others. This is not a click-download-install-go process, but a multi-step process that includes multiple programs and necessary configurations to make. Don’t worry! Both links above include detailed explanations for how to bring each piece online and go in to much greater detail than we can here. If you’ve completed the above, you’re ready to start developing in Ruby on Rails. Want to try setting up a blog? Enter the below in to the command terminal:$ rails new blog |
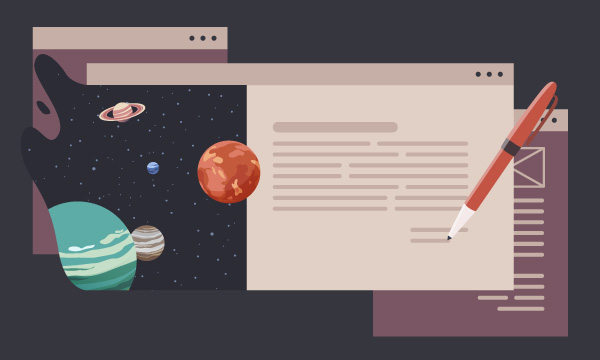
Next Steps
That new blog you created needs quite a lot more work before you show it off to anyone or even are able to use it yourself. Fortunately, Ruby on Rails makes building the basic functions of a blog—creating a page to post, managing the post themselves—simple enough. This Rails Guide on blog creation walks you through how to turn that barebones blog in to a fully fledged private publication. Ruby on Rails is a very action-oriented programming language, so most of the tutorials covering Ruby have you build something to learn the language. We’ve linked to a couple of them below:- How to build a login page through Ruby on Rails
- Building a bookmark manager through Ruby on Rails
- A Quora post with tutorials on how to build a chat application, an attendance record and a hangman game using Ruby on Rail
Reviewing code
Maybe you’ve written some Ruby code and have built a reasonably functional app or web interface. First, congrats on not having your project crash. But even if you haven’t created something that has produced a reprobative error message, it’s good practice to check your code. This will necessarily be part of your work once you start working with teams in you career, so it’s a good idea to explore the editing process early. But how?400 lines at a time
Many review guides have this suggested serving size written in. Why? Well, for one you’re going to get tired after reviewing more than 400 lines of code in one sitting. Also, you should spend no more than 90 minutes reviewing code to keep yourself alert. Research shows that the effectiveness of the code reviewer drops significantly after this amount of time.Adopt some good stylistic practice
Where possible, try to identify opportunities for you to simplify your code. Here are some helpful stylistic tips to help you make your code less messy.- Do not repeat yourself. If a line of code performs a function written elsewhere, eliminate one. This saves time when you’re revising your code as having to revise this function will involve checking two different points in the code. It sounds like a silly mistake to make, and you might promise yourself you’ll never make it until you see it in person. No worries! This is why we review our code.
- Delete unnecessary code. Remove unused variables. Clear out code the refers to objects that are no longer part of the project. Delete comments. Ruby on Rails will auto generate a whole project for you, and you may find that some parts of what automatically come out are unnecessary. Delete, delete, delete.
- Write discreet code. Try to write coding language that silos itself and works within the smallest scope possible. Try to keep objects decoupled and, where possible, keep your programming private or discreet to your specific contribution.
Explain your changes
Rarely will you starting from scratch when you get more involved in your coding career. Often, you’ll be working with functional code. Writing changes in to the code should include clear explanations about what was modified and why. So, as you write your new code in Ruby (or any other programming language) make sure you annotate it. Tip: do this after you’ve written your code so you have a chance to check your work before it makes it to your reviewer.Work within the system
Many firms likely have established protocols for working with changes in their source code. This Medium post from an iOS developer has a nice description of a typical Github change workflow, which includes a friendly graphic also. In summary, this process involves creating the source code, testing it, putting in a formal request for consideration, discussing the proposed change with the relevant staff, getting a thumbs up or down from them and them having your code incorporated pending approval.Keep a good attitude
Whether you’re reviewing your own code or reviewing code from other, catching a mistake shouldn’t be a reason to disparage either your own work or the work of others. Catching mistakes is what the editing process is for! Just like every good writer needs an effective editor to help them write publishable work, reviewing code is an important part of creating work that’s clean and usable.Find people
There are plenty of robust programming communities online that you ought to take advantage of. Coding can be a lonely activity and you should be focused on your work when you’re working, but find ways to grow through online communities and peer support. Platforms like Codeacademy enable coders to find one another in an online forum and provide technical, professional, and social support for eachother. An enterprising coder ought also to consider finding a mentor. Pairing yourself with someone more established in the trade can help you grow as a professional meaning you can take on harder tasks and (hopefully) command a higher premium. In short, don’t do it alone!Conclusion
Paired with Rails, Ruby is one of the of the most powerful programming tools available on the web today. It’s little wonder the programming language powers so much of the modern web, including Hulu and Basecamp. “But I’ve never coded before!” you may protest. As we’ve stated above, being a beginner is actually an asset to you here as Ruby is radically different from other programming languages. The Model-View-Controller scheme is one of a kind. But there’s no reason you’ll have to do it alone. Find the right people to support you and help you develop coding practices that work for you and eventually the team you’ll work with.
Tagged ruby developer